In this post, I am going to show you how to create a flow with Power Automate to break inheritance permissions on SharePoint List items or documents and add roles (new permissions). For that we are going to use the REST API of SharePoint.
Please note that I am writing this post on February 2020, it is possible that Power Automate has be enhanced and you might directly find an action to perform this task. However at the time I am writing this post, there is no other solution than using the SharePoint REST API.
You can also check how to break inheritance and manage permissions on List and Libraries (http://www.ludovicperrichon.com/power-automate-break-inherance-and-manage-roles-on-list-or-library/).
- Information: The SharePoint REST API
- Get groups and roles IDs
- Flow: Break inheritance on List items or documents
- Flow: Add roles/permissions on List items or documents
- Flow: Remove group on List items or documents
Information: The SharePoint REST API
The SharePoint REST API is used by developers in code solution, as we are going to use it in our flow, it is good to know how developers use this API to break ihnerance on List items or documents.
Don’t be affraid, I won’t get that much in details in the code and I will explain you step by step how to implement it with Power Automate.
So the REST API Code (under Javascript/jQuery code) to break inheritance roles and permissions on a List item or document is as following:
$.ajax({ url: "http://[MY_SHAREPOINT_URL]/_api/Lists/getById('[LIST_OR_LIBRARY_TITLE]')/items([ITEM_OR_DOCUMENT_ID])/breakroleinheritance(copyRoleAssignments=true,clearSubscopes=true)", type: "POST", headers: { "Accept": "application/json;odata=verbose", "content-Type": "application/json;odata=verbose", "X-RequestDigest": jQuery("#__REQUESTDIGEST").val() }, dataType: 'json', success: function (data) { // inheritance on list item broken }, error: function (error) { // Error in the process } });
As you can see, in the url I have “copyRoleAssignments=true”, if set to true, it means that it copy the roles/permissions of the parent, if set to false, it won’t copy the roles/permissions of the parent.
This is quite important to note, because if you add permissions, it will add a permissions, but others permissions won’t be replaced. That why I also show you how to remove permissions as well.
The REST API Code (under Javascript/jQuery code) to add roles/permissions on a List item or a document is as following:
$.ajax( { url: "http://[MY_SHAREPOINT_URL]/_api/web/lists/getByTitle('[LIST_OR_LIBRARY_TITLE]')/items([ITEM_OR_DOCUMENT_ID])/roleassignments/addroleassignment(principalid=[GROUP_ID],roleDefId=[ROLE_ID])", type: "POST", headers: { "Accept": "application/json;odata=verbose", "content-Type": "application/json;odata=verbose", "X-RequestDigest": jQuery("#__REQUESTDIGEST").val() }, dataType: 'json', success: function (data) { // Group and Role added }, error: function (error) { // Error in the process } });
You probably notice in the url that you need the group and the role ID to perform this task.
I will explain you how to get these IDs in Get groups and roles IDs.
The REST API Code (under Javascript/jQuery code) to remove a group permissions on a List item or a document is as following:
$.ajax( { url: "http://[MY_SHAREPOINT_URL]/_api/web/lists/getByTitle('[LIST_OR_LIBRARY_TITLE]')/items([ITEM_OR_DOCUMENT_ID])/roleAssignments/groups/removebyid([GROUP_ID])", type: "POST", headers: { "Accept": "application/json;odata=verbose", "content-Type": "application/json;odata=verbose", "X-RequestDigest": jQuery("#__REQUESTDIGEST").val() }, dataType: 'json', success: function (data) { // Group removed }, error: function (error) { // Error in the process } });
You probably notice in the url that you need the group ID to perform this task.
I will explain you how to get this ID in Get groups and roles IDs.
BE AWARE: When removing the group, it won’t have any access to the list item or the document, even Read permissions. You will have to add a read permission after removing for example if you need the users to have read access
TIPS: In these examples, I am getting the list with Title, in the flow I will use the Title as well, it can cause an issue if user change le Title of list, you can change the REST API call with the ID of list as following:
http://[MY_SHAREPOINT_URL]/_api/web/lists/getById('[LIST_OR_LIBRARY_GUID]')
Get groups and roles IDs
You can get these IDs through the SharePoint interface (can be a long process and role isn’t that simple) or by using PnP in PowerShell (faster and script below).
Using SharePoint interface
To get the group, you need to go to Site Permissions > Advanced Permissions settings > Click on the group you want the ID for > In the url:
[SHAREPOINT_URL]/_layouts/15/people.aspx?MembershipGroupId=5.
To get the role, it is a bit complicated by SharePoint interface, you can follow Ben Prins’s post to get the roles IDs.
Using PnP PowerShell
With PnP Powershell, it is quite easy to get the groups and roles ID. In the script below it will also pompt you the result as JSON, which will be needed for the Flow solution.
I have added a G_ to identify the groups names and a R_ to identify the roles names.
Connect-PnPOnline -Url "https://[MY_SHAREPOINT_URL]" -UseWebLogin Write-Host "{" $groups = Get-PnPGroup foreach($group in $groups){ Write-Host " `"G_$($group.Title)`" : $($group.Id)," } $roles = Get-PnPRoleDefinition $i = 0 foreach($role in $roles){ $i++ if($i -eq $roles.Count){ Write-Host " `"R_$($role.Name)`" : $($role.Id)" } else{ Write-Host " `"R_$($role.Name)`" : $($role.Id)," } } Write-Host "}"
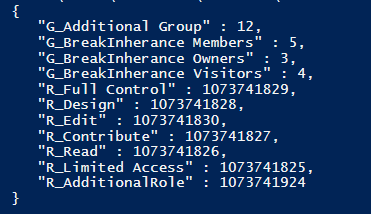
Flow: Break inheritance on List items or documents
For this flow, I will use a manual trigger. It is up to you to use another trigger if needed.
Add an Send HTTP Request to SharePoint action, and we will reproduce the API REST Call above.
For a document it is going to be the same process, put your Library title and the ID of your document item.
Example, on a list called Test and the item with ID 1:
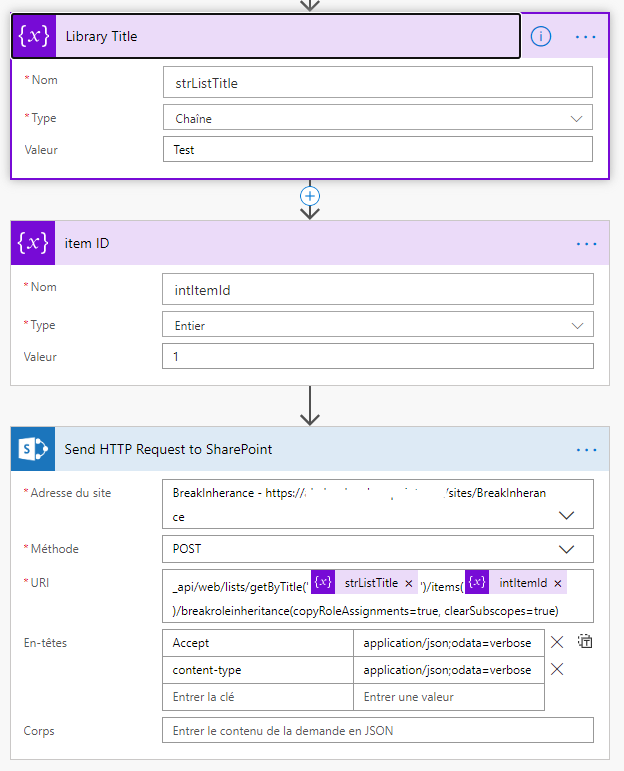
Of course, you can dynamically get item ID.
Need to Copy Paste?
Input | Value |
---|---|
URI | _api/web/lists/getByTitle(‘@{variables(‘strListTitle’)}’)/items(@{variables(‘intItemId’)})/breakroleinheritance(copyRoleAssignments=true, clearSubscopes=true) |
Accept | application/json;odata=verbose |
content-type | application/json;odata=verbose |
As mentionned in the Information: The SharePoint REST API part, the copyRoleAssignements if set to true, will copy the permissions of the parent. If set to false, it will erase all permissions.
Demo on a list item with copyRoleAssignements set to true: _api/web/lists/getByTitle(‘@{variables(‘strListTitle’)}’)/items(@{variables(‘intItemId’)})/breakroleinheritance(copyRoleAssignments=true, clearSubscopes=true)
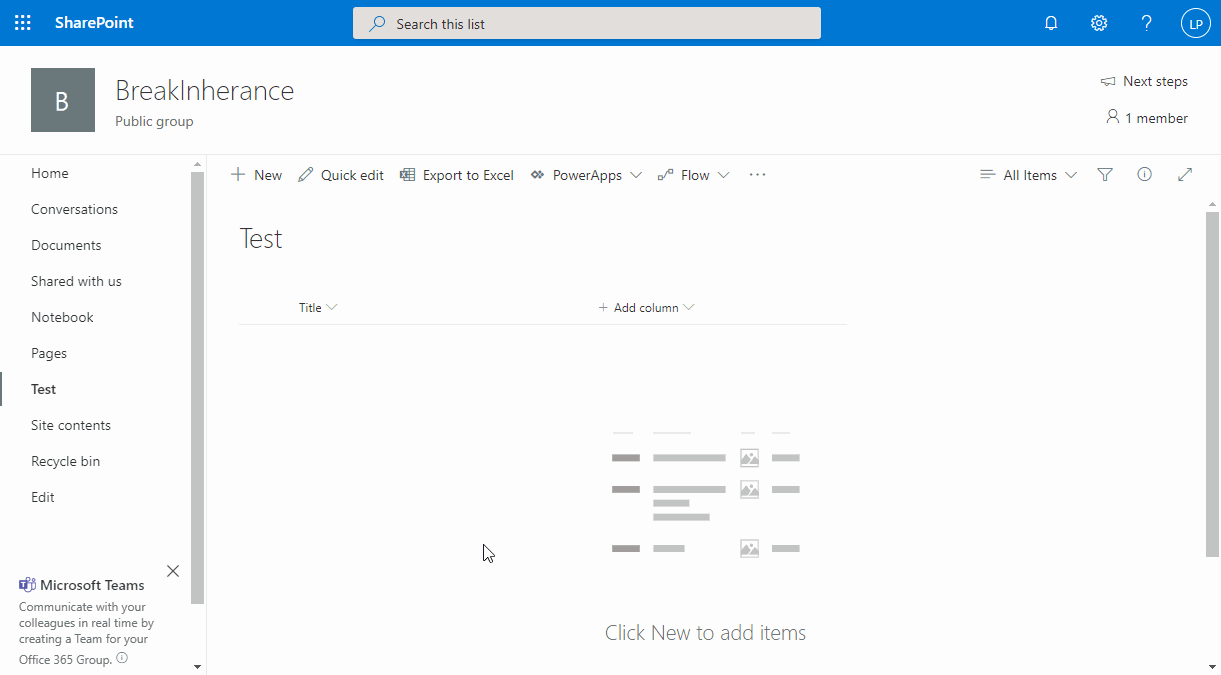
Demo on a list item with copyRoleAssignements set to false: _api/web/lists/getByTitle(‘@{variables(‘strListTitle’)}’)/items(@{variables(‘intItemId’)})/breakroleinheritance(copyRoleAssignments=false, clearSubscopes=true)
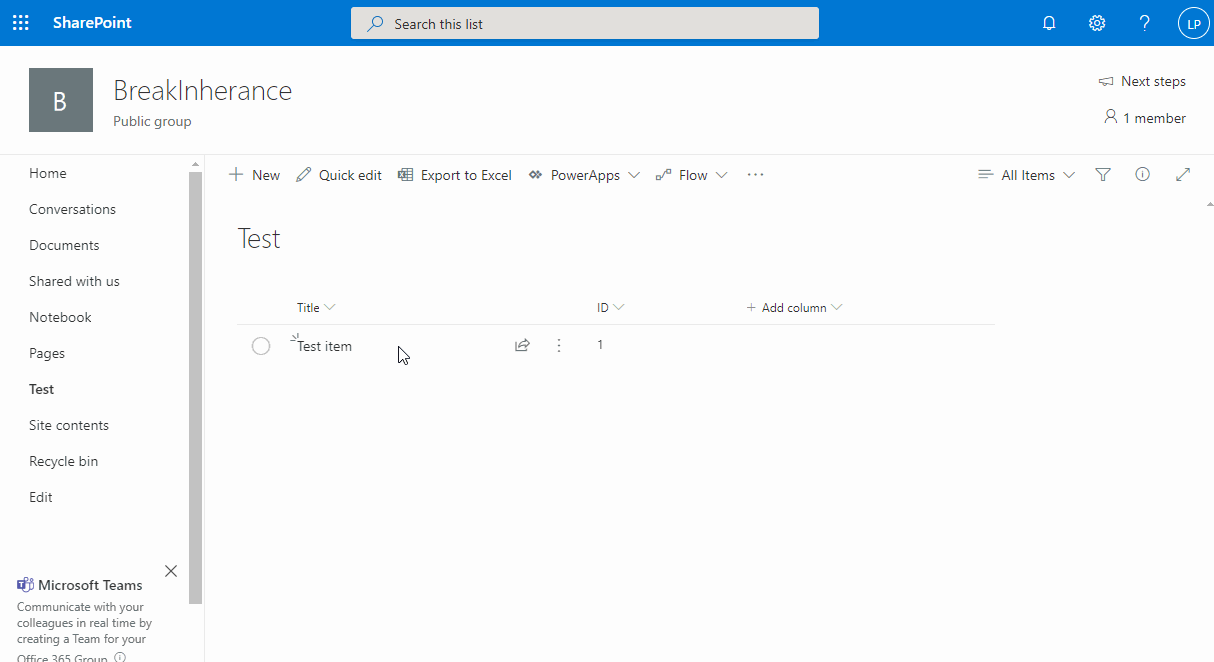
Flow: Add roles/permissions on List items or documents
For this flow, I will use a manual trigger. It is hope to you to use another trigger if needed.
Pre-requisite: Your list item or document where you are going to add permissions, need to have inheritance permissions broken. See above.
Firstable I will initialize a JSON containing all my IDs, you can initialize each ID in a variable, but that means you are going to initialize as many variables as there are IDs.
Also please note, I have created an additional group and an additional permission for the demo.
If you use PnP, you just need to copy paste the JSON prompted in the console.
Otherwise create your JSON as following:
{ "MY GROUP OR ROLE NAME TO BE IDENTIFY IN FLOW" : GROUP_OR_ROLE_ID }
Add a Parse JSON action, copy the JSON in Content, click on generate schema, copy your JSON and click on Done.
Demo below is in French, but don’t worry, I change the name with English one and buttons and fields are at the same place.
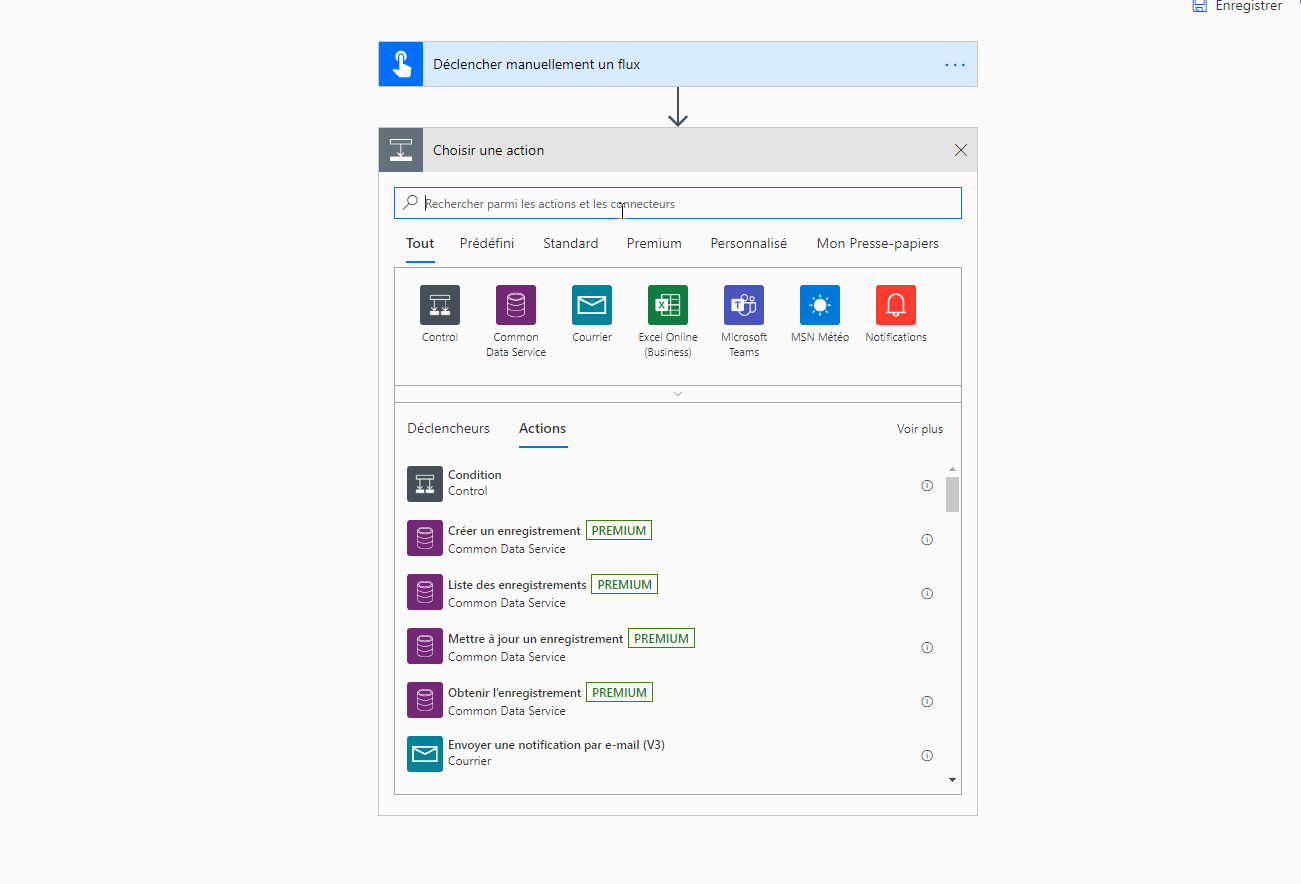
Then add an action Send HTTP Request to SharePoint, we are going to reproduce the API Rest call defined in Information: The SharePoint REST API.
Here I am going to give Members group the read permissions on my list item with ID 1. If you want to perform it on Document, just change list title by your library title and put your document ID.
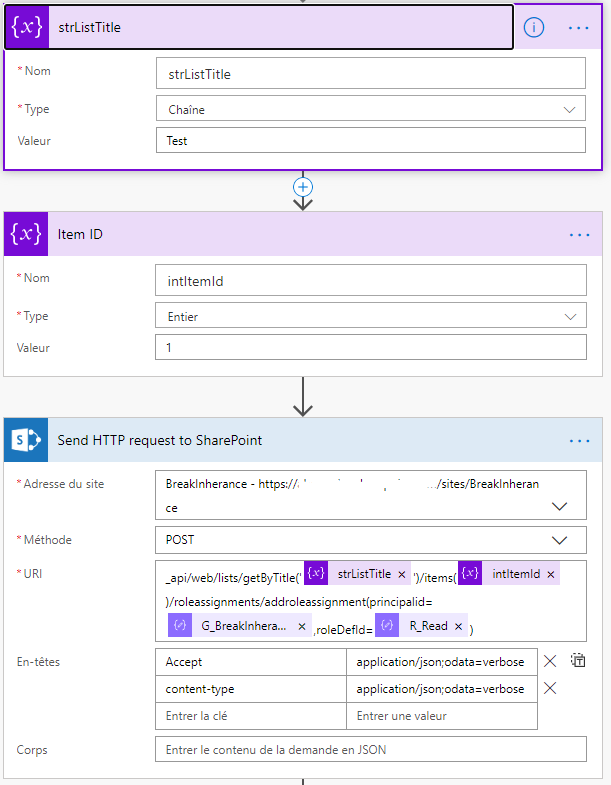
Need to Copy Paste?
Input | Value |
---|---|
URI | _api/web/lists/getByTitle(‘@{variables(‘strListTitle’)}’)/items(@{variables(‘intItemId’)})/roleassignments/addroleassignment(principalid=@{body(‘Parse_JSON_:_IDs’)?[‘G_BreakInherance Members’]},roleDefId=@{body(‘Parse_JSON_:_IDs’)?[‘R_Read’]}) |
Accept | application/json;odata=verbose |
content-type | application/json;odata=verbose |
If you need to change more permissions, just add another Send HTTP request to SharePoint.
Example, I am adding permissions to my additional group with the additional role.
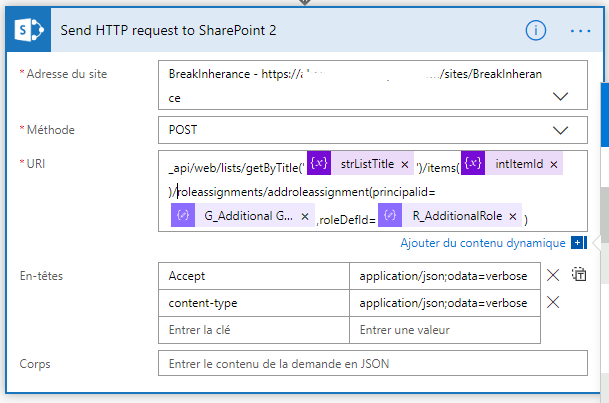
Demo:
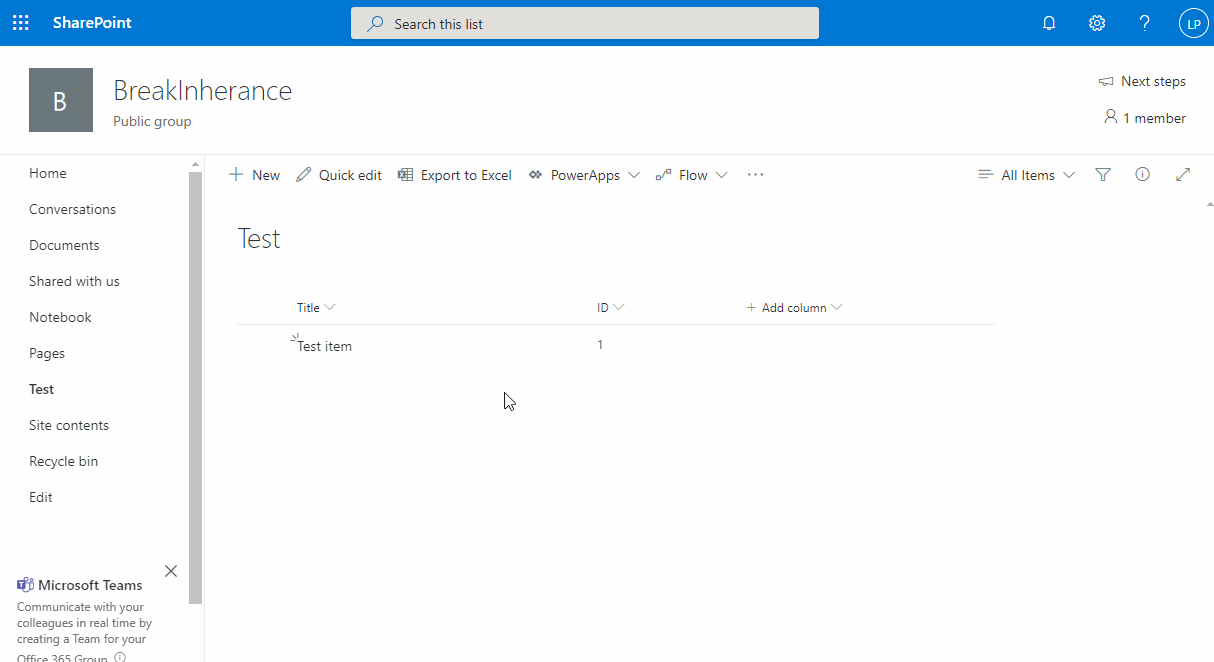
IMPORTANT: If your group already have permissions, it will add the new permissions to the existing one. For my example in my case, I am adding Read permissions on the list item, if the members already have Edit permissions, it won’t remove the Edit one, you will see that he have Edit and Read permissions

Flow: Remove group on List items or documents
For this flow, I will use a manual trigger. It is hope to you to use another trigger if needed.
Pre-requisite: Your list item or document where you are going to remove permissions, need to have inheritance permissions broken. See above.
Firstable I will initialize a JSON containing all my IDs, you can initialize each ID in a variable, but that means you are going to initialize as many variables as there are IDs.
Also please note, I have created an additional group and an additional permission for the demo.
If you use PnP, you just need to copy paste the JSON prompted in the console.
Otherwise create your JSON as following:
{ "MY GROUP OR ROLE NAME TO BE IDENTIFY IN FLOW" : GROUP_OR_ROLE_ID }
Add a Parse JSON action, copy the JSON in Content, click on generate schema, copy your JSON and click on Done.
Demo below is in French, but don’t worry, I change the name with English one and buttons and fields are at the same place.
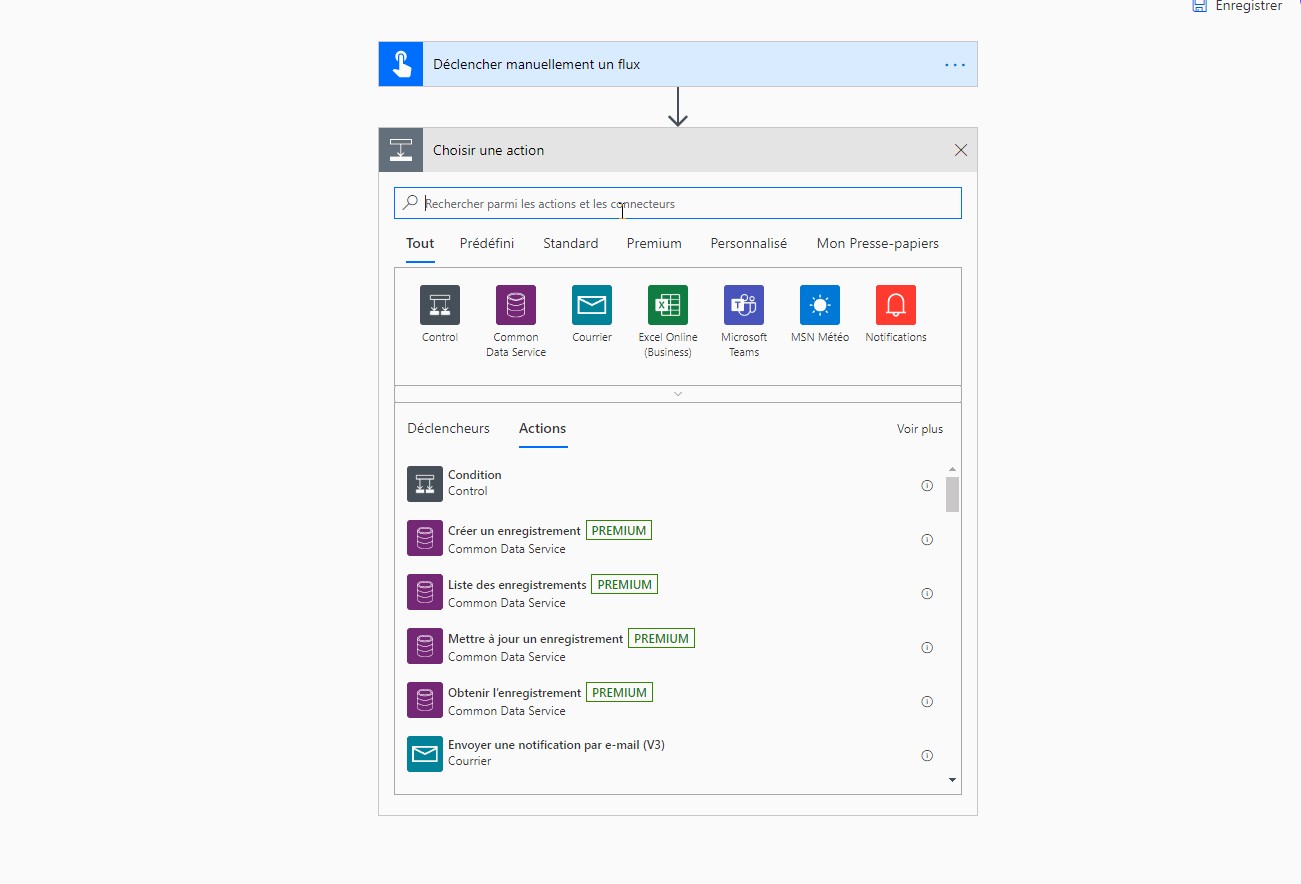
Then add an action Send HTTP Request to SharePoint, we are going to reproduce the API Rest call defined in Information: The SharePoint REST API.
Here I am going to remove the Members group from my item ID 1.
If you want to perform it on Document, just change list title by your library title and put your document ID.
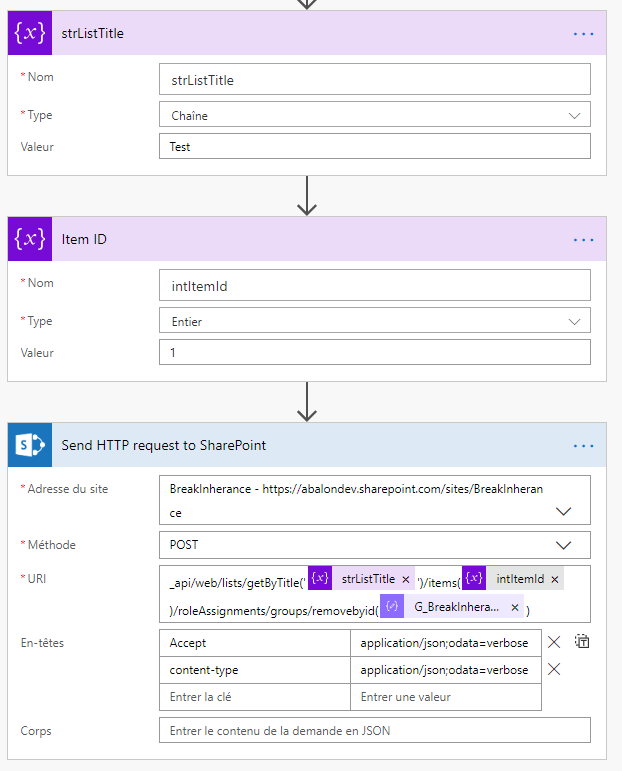
Need to Copy Paste?
Input | Value |
---|---|
URI | _api/web/lists/getByTitle(‘@{variables(‘strListTitle’)}’)/items(@{variables(‘intItemId’)})/roleAssignments/groups/removebyid(@{body(‘Parse_JSON_:_IDs’)?[‘G_BreakInherance Members’]}) |
Accept | application/json;odata=verbose |
content-type | application/json;odata=verbose |
Demo:
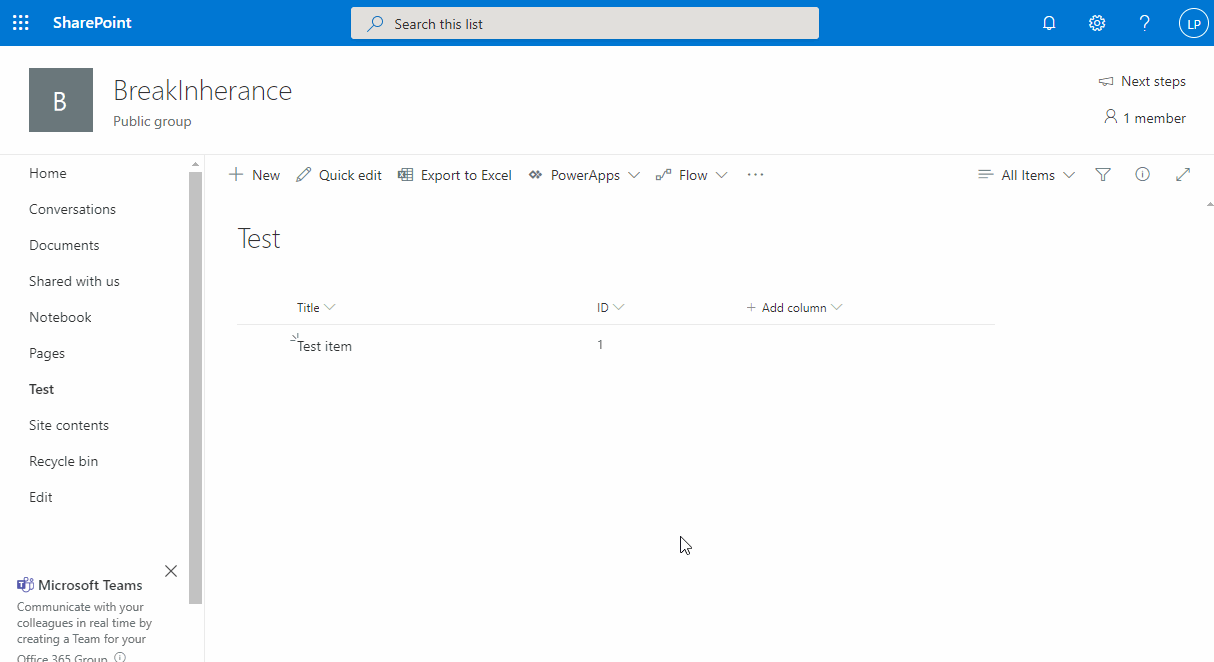
You can also check how to break inheritance and manage permissions on List and Libraries (http://www.ludovicperrichon.com/power-automate-break-inherance-and-manage-roles-on-list-or-library/).